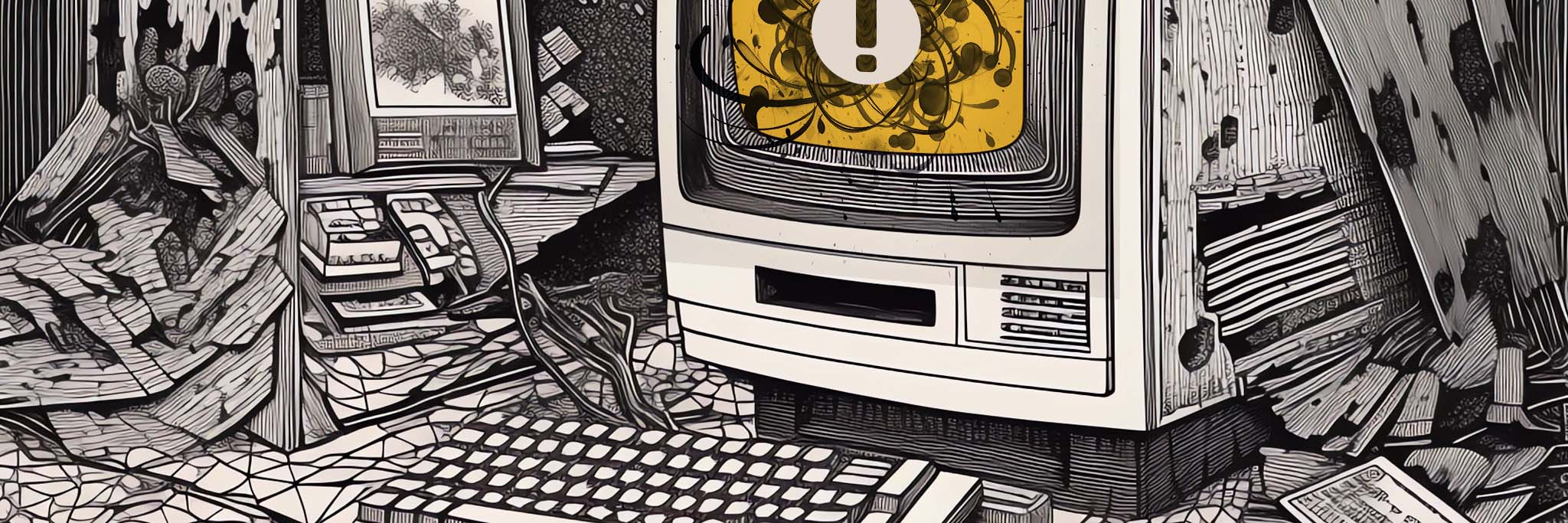
React Error #130
Are you experiencing a cryptic error in your console saying React Error #130? Or often something like “Error: Minified React error #130” ?
When working with React (For me, while working with it in WordPress development), this error often appears with little or no explanation as to what’s wrong, what you need to change, or even what file it is coming from.
There are lots of stack overflow pages about this error that jump headfirst into detail, so here I want to describe at a high level some of the very basic mistakes that can be causes.
The first thing you might find, is simply that you don’t even know where to begin. More specifically, what file is causing it. So let’s start there.
The issue
In my experience, this error is usually caused by react being unable to find a component. More specifically, you’ve imported a class from one file into another while the code is logically sound within each file, there’s an error in the link between them.
Incorrect naming
Start by checking the naming. If you’ve created a class called MyButton
and then tried to import it using the name MyButtons then react won’t be able to find it.
Incorrect import format
If naming isn’t the culprit, then check that you’ve imported it in a way that matches the way you have exported it. There are two forms of exporting that you’ll need to be aware of: Named Exports, and Default Exports.
Default export
You can only have one default export in a file, and when you’ll need to have defined it with the keywords export default like this:
export default class CustomClass extends React.Component {
...
}
And then in the file where you import, you import by simply giving it a name and saying what it’s from. Here I’ve used the same name, but because the file knows which one is default, I can put any name here:
import CustomClass from './CustomClass.jsx';
Named export
Alternatively, with a named export, you can have any number of exports in the source file. For named exports you simply use the keyword export and name that’s assigned will be the same as the name of the class, function, or variable automatically.
Here’ we can see’s an example of 2 named exports called CustomClass1 and CustomClass2.
export class CustomClass1 extends React.Component {
...
}
export class CustomClass2 extends React.Component {
...
}
When you import those in another file, however, you must place them in curly braces and separated by commas. Which is different to the default exports.
And like in this example, you must use the exact names assigned to them in the source file:
import { CustomClass1, CustomClass2 } from './CustomClasses.jsx';
Hopefully that’s helped you figure out where you’ve gone wrong that’s causing the error. If you’ve tried to import the file incorrectly, then react won’t be able to find it and will give you the error.
It’s also worth noting that the above types of exports can also be done at the same time like in the below example. And that’s completely valid, but check each classes import is consistent with how it was defined.
export default class CustomClass extends React.Component {
...
}
export class CustomClass1 extends React.Component {
...
}
export class CustomClass2 extends React.Component {
...
}
import CustomClass, { CustomClass1, CustomClass2 } from './CustomClasses.jsx';
Finding the problem file
If you haven’t done much error debugging, here are a couple of tips to help.
If you change 20 files before you actually test your project, then this error could be coming from any of them and you’ll have to try and narrow it down or check all of them. So it’s good to have a habit of running your project regularly. That way, you’ll likely have changed less files since last time you ran it, and so when you notice the error you’ll only have to check 2 or 3 files.
If you’re unsure which files you’ve changed, and you’re using GIT, run git status
in your terminal to see which files have changed, or check Visual Studio Code’s Source Control tab. It will show you which files were changed since your last commit, which will hopefully help you narrow it down. And clicking on them will also show the lines that changed, which is very helpful too.
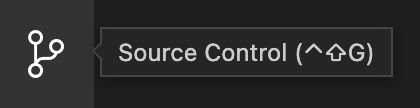
That’s it!
I’d love to know if any of these articles helped you.
Share what you’re building or ask me a question on Threads or somewhere else.
Instagram is a great place to see my final creative coding experiments, while the others are are great place to connect or see progress updates.
If my content has helped you, I’d never say no to donations to help me keep writing.
Here are some other things you might like
- Extracting the extension from a filename string
- Register a new file type in Obsidian
- Transparent React Native iFrames/WebViews
Leave a Reply