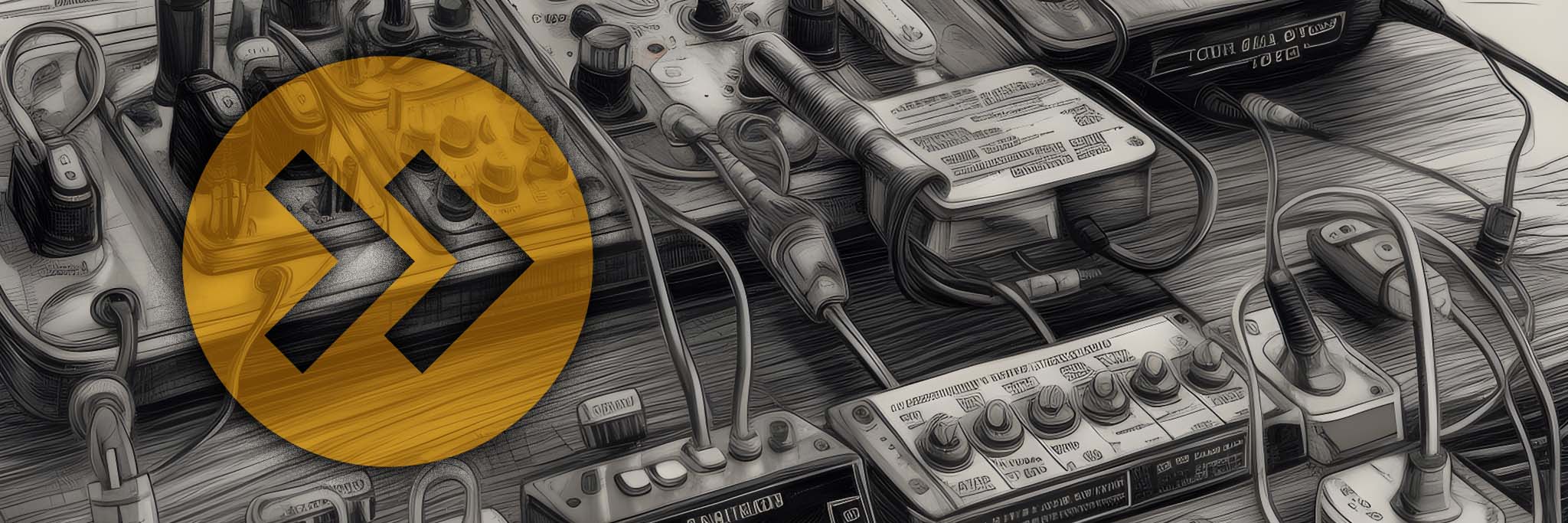
A Basic Custom esbuild Rename Plugin
Recently, in a project using esbuild, I found myself wanting to rename a file after the build process finished.
The problem was, I was using esbuild-sass-plugin to compile the scss files scattered around my project into a single css file, and I couldn’t figure out how to customise the output filename that it used. This may have been a lack of knowledge on my part, but either way, it output a file called main.css that I needed to be styles.css.
So… I wrote myself a super basic esbuild plugin that simply waits until the build has finished and then renames the output file. Not the most elegant solution but one that worked to get me back to working on my actual project.
You’ll find the code below.
The plugin code
Below is all of the plugin’s code, which is hardcoded to simply rename the file main.css to styles.css.
This was a requirement for me because the project is a plugin for Obsidian, and in that ecosystem, plugins require a styles.css file in the final output.
import fs from 'fs';
const renamePlugin = () => ({
name: 'rename-plugin',
setup(build) {
build.onEnd(async () => {
try {
fs.renameSync('./dist/main.css', './dist/styles.css');
} catch (e) {
console.error('Failed to rename file:', e);
}
});
},
});
Using the plugin
To use the above code, I wrote it directly in my esbuild.config.mjs like in the screenshot below, however, you could also put it in a separate file and import it in.
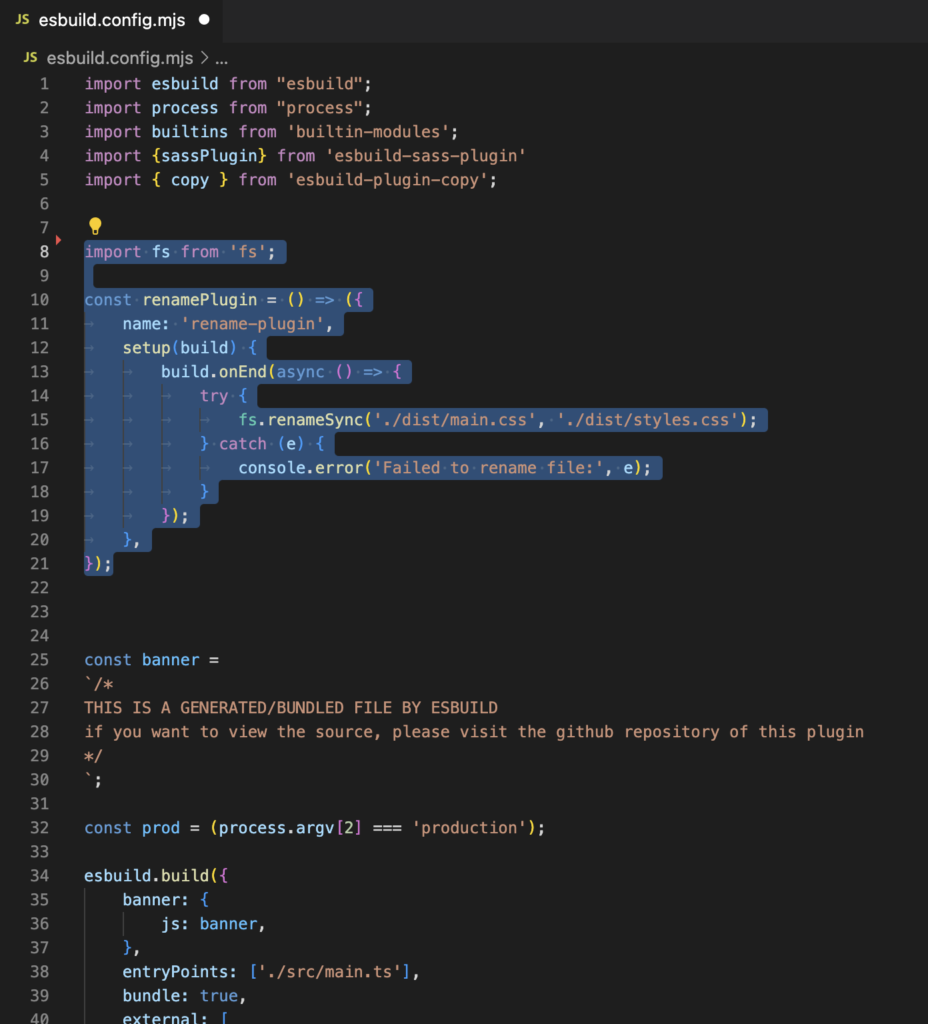
To call the plugin on build, it’s just like calling any other plugin, but since it’s functionality is hard coded, it has no settings.
esbuild.build({
...
plugins: [
...
renamePlugin(),
],
})
Remember: Because this plugin renames a file after it get’s compiled, it has to be at the end of the plugin’s list or the file it’s looking to rename won’t exist yet.
That’s it!
While there may be other ways to solve this, hopefully it showed you that custom esbuild plugins can be pretty easy and handy, and maybe it even got you unstuck like it did for me.
I’d love to know if any of these articles helped you.
Share what you’re building or ask me a question on Threads or somewhere else.
Instagram is a great place to see my final creative coding experiments, while the others are are great place to connect or see progress updates.
If my content has helped you, I’d never say no to donations to help me keep writing.
Here are some other things you might like
- Extracting the extension from a filename string
- Register a new file type in Obsidian
- Transparent React Native iFrames/WebViews
Leave a Reply